Mastering Nodemailer | Send Emails Like a Pro in Your Node.js App | AV Coding
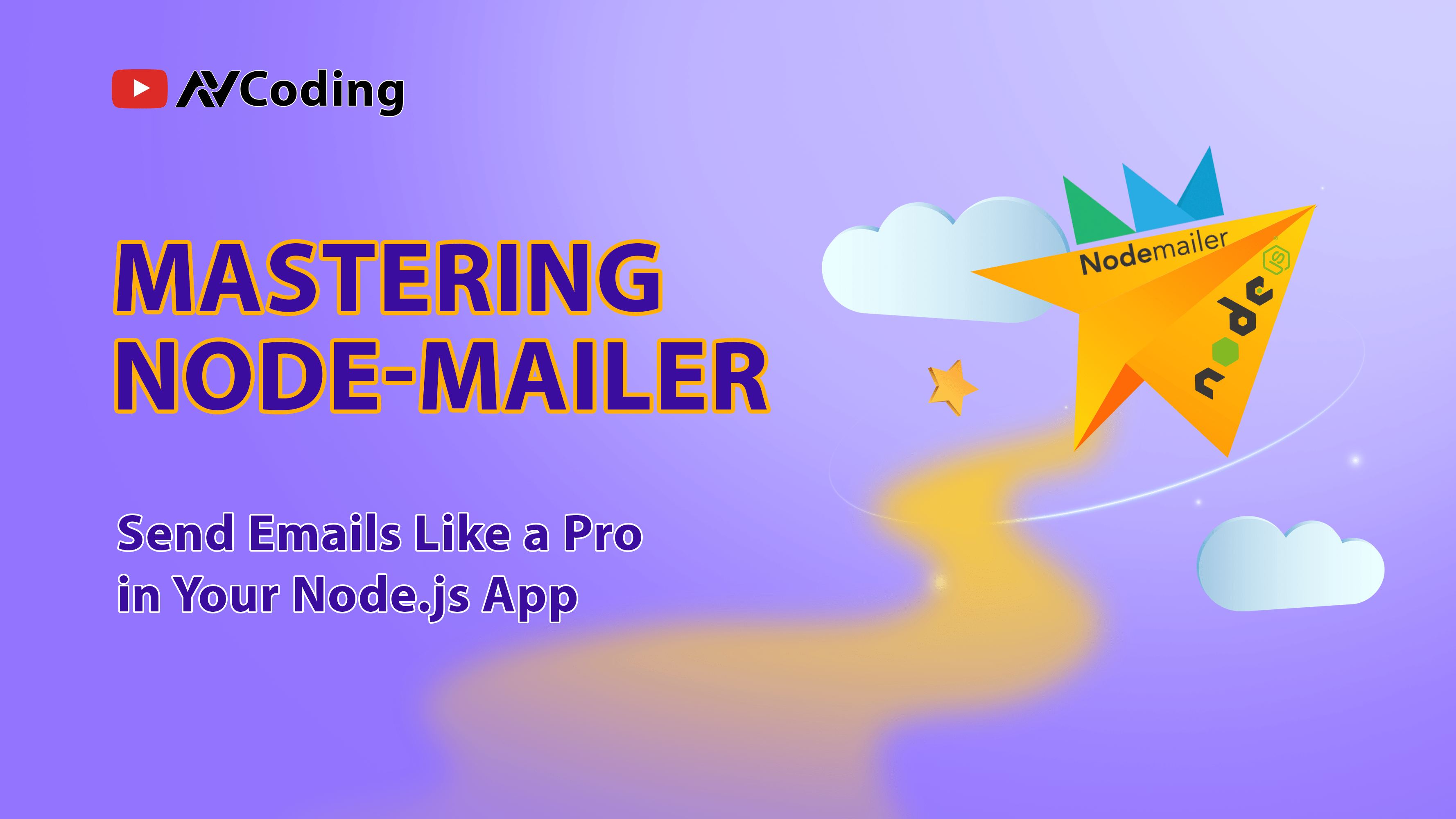
Hey guys welcome to AV Coding
Today, we will delve into how to integrate the node-mailer library in node js.
NPM REPO: nodemailer – npm (npmjs.com)
Node-Mailer Doc: Nodemailer :: Nodemailer
mailer.js
var express = require('express');
var router = express.Router();
const nodemailer = require("nodemailer");
const util = require('util');
const transporter = nodemailer.createTransport({
sendMail: true,
name: "mail.ashutoshviramgama.com",
host: "mail.ashutoshviramgama.com",
port: 465,
pool: true,
secure: true,
auth: {
user: "node-mailer@ashutoshviramgama.com",
pass: "pwd",
},
debug: true,
// SES: { ses, aws },
// dkim: {
// domainName: "example.com",
// keySelector: "2017",
// privateKey: "-----BEGIN PRIVATE KEY-----\nMIIEvgIBADANBg..."
// },
// auth: {
// type: 'custom',
// method: 'MY-CUSTOM-METHOD', // forces Nodemailer to use your custom handler
// user: 'username',
// pass: 'verysecret'
// },
// customAuth: {
// 'MY-CUSTOM-METHOD': ctx => {
// let cmd = await ctx.sendCommand(
// 'AUTH PLAIN ' +
// Buffer.from(
// '\u0000' + ctx.auth.credentials.user + '\u0000' + ctx.auth.credentials.pass,
// 'utf-8'
// ).toString('base64')
// );
// cmd.status
// cmd.code
// cmd.text
// cmd.response
// if(cmd.status < 200 || cmd.status >= 300){
// throw new Error('Failed to authenticate user: ' + cmd.text);
// }
// }
// },
// proxy: 'socks5://socks-host:1234',
});
// enable support for socks URLs
// transporter.set('proxy_socks_module', require('socks'));
// custom proxy handler
// transporter.set('proxy_handler_myproxy', handler);
// transporter.set('proxy_handler_myproxy', (proxy, options, callback) => {
// console.log('Proxy host=% port=%', proxy.hostname, proxy.port);
// let socket = require('net').connect(options.port, options.host, () => {
// callback(null, {
// connection: socket
// });
// });
// });
// verify connection configuration
transporter.verify(function (error, success) {
if (error) {
console.log(error);
} else {
console.log("Server is ready to take our messages");
}
});
router.get('/', function (req, res, next) {
transporter.sendMail({
from: '"AV Coding 👻" <node-mailer@dummy.com>',
to: "ashutoshviramgama@gmail.com, dummy2@gmail.com",
cc: "dummy@gmail.com",
bcc: [
"dummy@gmail.com",
{
name: "Ashutosh Viramgama",
address: "dummy@gmail.com"
}
],
subject: "Hello This is Node Test Mailer from AV Coding ✔",
headers: {
'x-my-key': 'header value',
'x-another-key': 'another value'
},
text: "Hello to AV Coding world?",
html: `Hello AV Coding world? Embedded image:
`,
icalEvent: {
filename: 'invitation.ics',
method: 'request',
content: "BEGIN:VCALENDAR\r\nPRODID:-//ACME/DesktopCalendar//EN\r\nMETHOD:REQUEST\r\n..."
},
attachments: [
{ // utf-8 string as an attachment
filename: 'text1.txt',
content: 'hello world!'
},
{ // binary buffer as an attachment
filename: 'text2.txt',
content: new Buffer('hello world!', 'utf-8')
},
{ // file on disk as an attachment
filename: 'text3.txt',
path: 'https://picsum.photos/id/1/200/300' // stream this file
},
{ // filename and content type is derived from path
href: 'https://picsum.photos/id/1/200/300'
},
// { // stream as an attachment
// filename: 'text4.txt',
// content: fs.createReadStream('file.txt')
// },
{ // define custom content type for the attachment
filename: 'text.bin',
content: 'hello world!',
contentType: 'text/plain'
},
{ // use URL as an attachment
filename: 'license.txt',
path: 'https://raw.github.com/nodemailer/nodemailer/master/LICENSE'
},
{ // encoded string as an attachment
filename: 'text1.txt',
content: 'aGVsbG8gd29ybGQh',
encoding: 'base64'
},
{ // data uri as an attachment
path: 'data:text/plain;base64,aGVsbG8gd29ybGQ='
},
{
// use pregenerated MIME node
raw: 'Content-Type: text/plain\r\n' +
'Content-Disposition: attachment;\r\n' +
'\r\n' +
'Hello world!'
},
{
filename: 'image.png',
path: 'https://picsum.photos/id/1/200/300',
cid: 'unique@nodemailer.com' //same cid value as in the html img src
}
],
alternatives: [
{
contentType: 'text/x-web-markdown',
content: '**Hello AV Coding world!**'
}
],
list: {
// List-Help: <mailto:admin@example.com?subject=help>
help: 'node-mailer@ashutoshviramgama.com?subject=help',
// List-Unsubscribe: <http://example.com> (Comment)
unsubscribe: {
url: 'https://ashutoshviramgama.com',
comment: 'Comment'
},
// List-Subscribe: <mailto:admin@example.com?subject=subscribe>
// List-Subscribe: <http://example.com> (Subscribe)
subscribe: [
'node-mailer@ashutoshviramgama.com?subject=subscribe',
{
url: 'https://ashutoshviramgama.com',
comment: 'Subscribe'
}
],
// List-Post: <http://example.com/post>, <mailto:admin@example.com?subject=post> (Post)
post: [
[
'https://ashutoshviramgama.com/post',
{
url: 'node-mailer@ashutoshviramgama.com?subject=post',
comment: 'Post'
}
]
]
},
dsn: {
id: 'ThisIsFirstTimeForAVCOding_01',
return: 'headers',
notify: ['failure', 'delay', 'success', 'never'],
recipient: 'node-mailer@ashutoshviramgama.com'
},
// envelope: {
// from: 'AV Coding <node-mailer@ashutoshviramgama.com>', // used as MAIL FROM: address for SMTP
// to: 'ashutoshviramgama@gmail.com, Mailer <ashutoshviramgama@gmail.com>' // used as RCPT TO: address for SMTP
// },
// raw: `From: node-mailer@ashutoshviramgama.com
// To: ashutoshviramgama@gmail.com
// Subject: AV Coding test message
// Hello AV Coding world!`,
}).then(info => {
res.status(200).send("Message sent: " + util.inspect(info, { depth: null }));
}).catch(err => {
console.log('Preview Error: ' + err);
res.status(404).send("Message Error: " + util.inspect(err, { depth: null }));
});
});
module.exports = router;
output of info
{
accepted: [ 'ashutoshviramgama@gmail.com', 'dummy@gmail.com' ],
rejected: [],
ehlo: [
'SIZE 52428800',
'8BITMIME',
'PIPELINING',
'PIPECONNECT',
'AUTH PLAIN LOGIN',
'HELP'
],
envelopeTime: 57,
messageTime: 32,
messageSize: 166,
response: '250 OK id=1s0dfz-004NY8-3A',
envelope: {
from: 'node-mailer@ashutoshviramgama.com',
to: [ 'ashutoshviramgama@gmail.com', 'dummy@gmail.com' ]
},
messageId: '<3c7ea9ee-305c-e960-7809-cfaf771a3afc@ashutoshviramgama.com>'
}
Hope You Liked This Blog. Share, Comment, Subscribe for More Code Feeds
0 Comments