How To Show Dynamic Timer On Websites | AV Coding
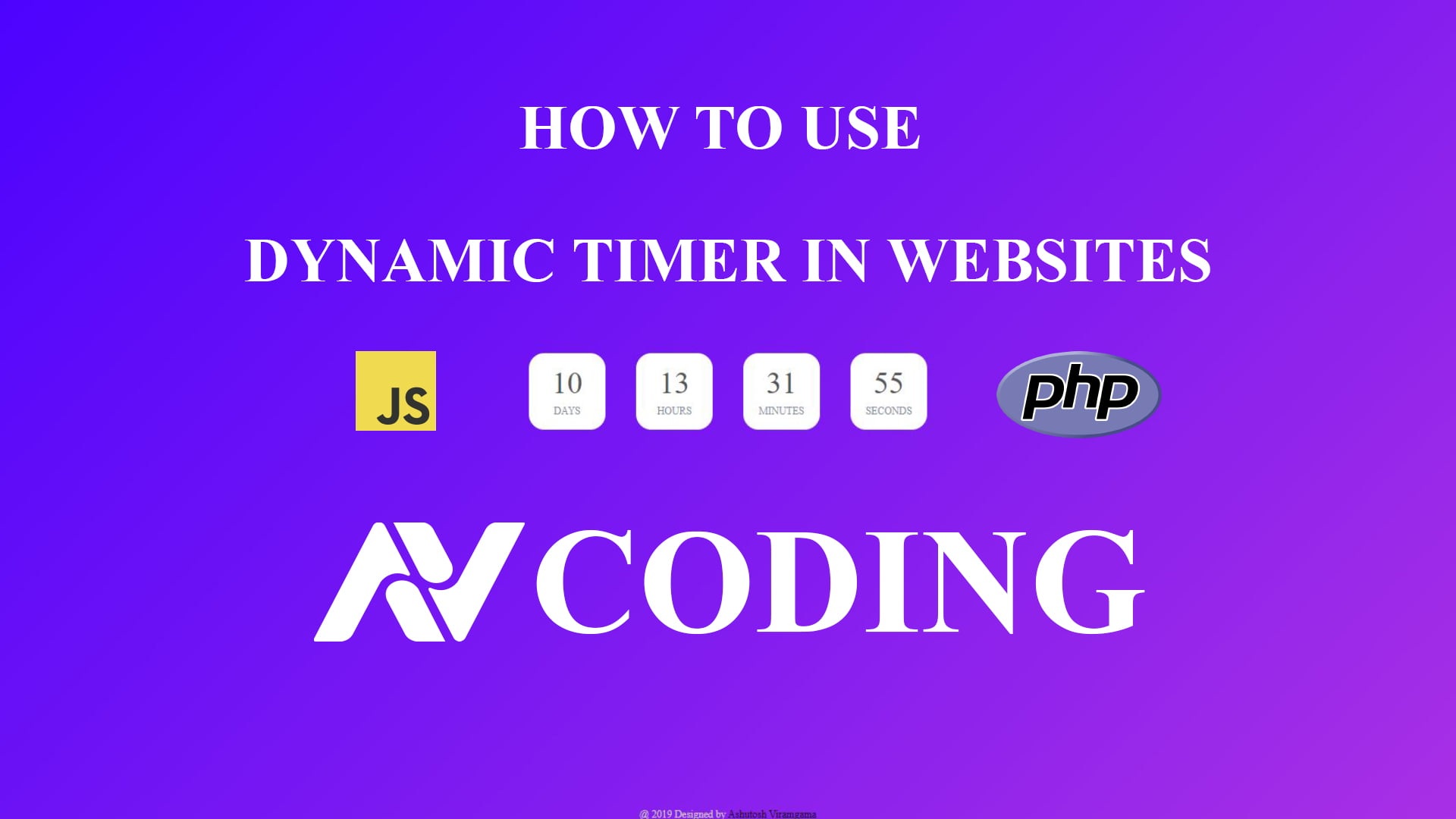
Hey guys welcome to AV Coding
Today we will learn how to show dynamic timer on website using php and JavaScript.
The complete code is explained in the video below and the source code is provided below.
<?php
// define times to countdown to and the time now
$time1 = strtotime(gmdate("2020/02/18"));
$time2 = "";
$time3 = "";
$time4 = "";
$timeNow = strtotime("now");
?>
<!DOCTYPE html>
<html lang="en">
<head>
<title>AV Coding</title>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!--===============================================================================================-->
<link rel="icon" type="image/png" href="https://ashutoshviramgama.com/wp-content/uploads/2018/12/cropped-logo-192x192.png"/>
<!--===============================================================================================-->
<link rel="stylesheet" type="text/css" href="https://onstechhub.com/vendor/bootstrap/css/bootstrap.min.css">
<!--===============================================================================================-->
<link rel="stylesheet" type="text/css" href="https://onstechhub.com/fonts/font-awesome-4.7.0/css/font-awesome.min.css">
<!--===============================================================================================-->
<link rel="stylesheet" type="text/css" href="https://onstechhub.com/fonts/iconic/css/material-design-iconic-font.min.css">
<!--===============================================================================================-->
<link rel="stylesheet" type="text/css" href="https://onstechhub.com/vendor/animate/animate.css">
<!--===============================================================================================-->
<link rel="stylesheet" type="text/css" href="https://onstechhub.com/vendor/select2/select2.min.css">
<!--===============================================================================================-->
<link rel="stylesheet" type="text/css" href="https://onstechhub.com/css/util.css">
<link rel="stylesheet" type="text/css" href="https://onstechhub.com/css/main.css">
<!--===============================================================================================-->
</head>
<body>
<div class="bg-g1 size1 flex-w flex-col-c-sb p-l-15 p-r-15 respon1">
<span></span>
<div class="flex-col-c">
<img class='mb-5' src="https://ashutoshviramgama.com/wp-content/uploads/2020/02/logo.png" height="150">
<div class="flex-w flex-c cd100">
<div class="flex-col-c-m size2 how-countdown">
<span class="l1-txt3 p-b-9 days" id="days"></span>
<span class="s1-txt1">Days</span>
</div>
<div class="flex-col-c-m size2 how-countdown">
<span class="l1-txt3 p-b-9 hours" id="hours"></span>
<span class="s1-txt1">Hours</span>
</div>
<div class="flex-col-c-m size2 how-countdown">
<span class="l1-txt3 p-b-9 minutes" id="minutes"></span>
<span class="s1-txt1">Minutes</span>
</div>
<div class="flex-col-c-m size2 how-countdown">
<span class="l1-txt3 p-b-9 seconds" id="seconds"></span>
<span class="s1-txt1">Seconds</span>
</div>
</div>
</div>
<span class="s1-txt3 txt-center">
@ 2019 Designed by <a style="color: black;" href="https://ashutoshviramgama.com/" target="_blank">Ashutosh Viramgama</a>
</span>
</div>
<!--===============================================================================================-->
<!-- <script src="vendor/jquery/jquery-3.2.1.min.js"></script> -->
<!--===============================================================================================-->
<!-- <script src="vendor/bootstrap/js/popper.js"></script> -->
<!-- <script src="vendor/bootstrap/js/bootstrap.min.js"></script> -->
<!--===============================================================================================-->
<!-- <script src="vendor/select2/select2.min.js"></script> -->
<!--===============================================================================================-->
<!-- <script src="vendor/countdowntime/moment.min.js"></script>
<script src="vendor/countdowntime/moment-timezone.min.js"></script>
<script src="vendor/countdowntime/moment-timezone-with-data.min.js"></script>
<script src="vendor/countdowntime/countdowntime.js"></script> -->
<script>
var time1 = parseInt("<?php echo $time1;?>");
var time2 = parseInt("<?php echo $time2;?>");
var time3 = parseInt("<?php echo $time3;?>");
var time4 = parseInt("<?php echo $time4;?>");
var timeNow = parseInt("<?php echo $timeNow;?>");
// difference in seconds between highest PM time and lowest AM time
var highLowDif = 21600;
// create an array to store times in
var timeInSeconds = [ time1, time2, time3, time4 ];
// initiate the target hour variable
var targetHour = 0;
// loop through each element and retrieve the next time to countdown to
for(var i = 0; i < timeInSeconds.length; i++) {
// if this time is greater than the current time assign it to targetHour variables and break out of
// the loop because we only want the next highest
if(timeInSeconds[i] > timeNow) {
targetHour = timeInSeconds[i];
break;
}
// If there is no higher time than get the next lowest time
var temp = timeInSeconds[i];
if(timeInSeconds[i] > temp){
temp = TimeInSeconds[i];
}
}
// If no higher time could be found then its really late at night and the next time is in the am
if(targetHour == 0){
// get difference between time now and the earliest am time
var highDif = timeNow - temp;
// to count down from late pm to early am we find out how many seconds it is between now and the next
// time, subtract the difference from this number and then add the remainder to the time now
targetHour = timeNow + (highLowDif - highDif);
}
// get the difference between the next time and the time now to work out how long there is to go in seconds
var dif = targetHour - timeNow;
// run the timer
var counter=setInterval(timer, 1000); //1000 will run it every 1 second
function timer()
{
// if we reach the target time then restart the timer for the next time we want to count down to
if(dif == 0){
clearInterval(counter);
}else{
// work out how many hours, minutes and seconds there are
var dy1 = Math.floor(dif/(3600*24)) % 60;
var dh1 = Math.floor(dif/3600) % 60;
var dm1 = Math.floor(dif/60) % 60;
var ds1 = dif % 60;
// countdown one second
dif--;
// show current time left on the countdown timer
document.getElementById("seconds").innerHTML=ds1;
document.getElementById("days").innerHTML=dy1;
document.getElementById("minutes").innerHTML=dm1;
document.getElementById("hours").innerHTML=dh1;
}
}
</script>
<!--===============================================================================================-->
<!-- <script src="vendor/tilt/tilt.jquery.min.js"></script>
<script >
$('.js-tilt').tilt({
scale: 1.1
})
</script> -->
<!--===============================================================================================-->
<!-- <script src="js/main.js"></script> -->
</body>
</html>
Hope You Liked This Blog. Share, Comment, Subscribe And Press The Bell Icon In The Bottom Right Side For More Code Feeds.
0 Comments