Dynamic Hierarchy Logic
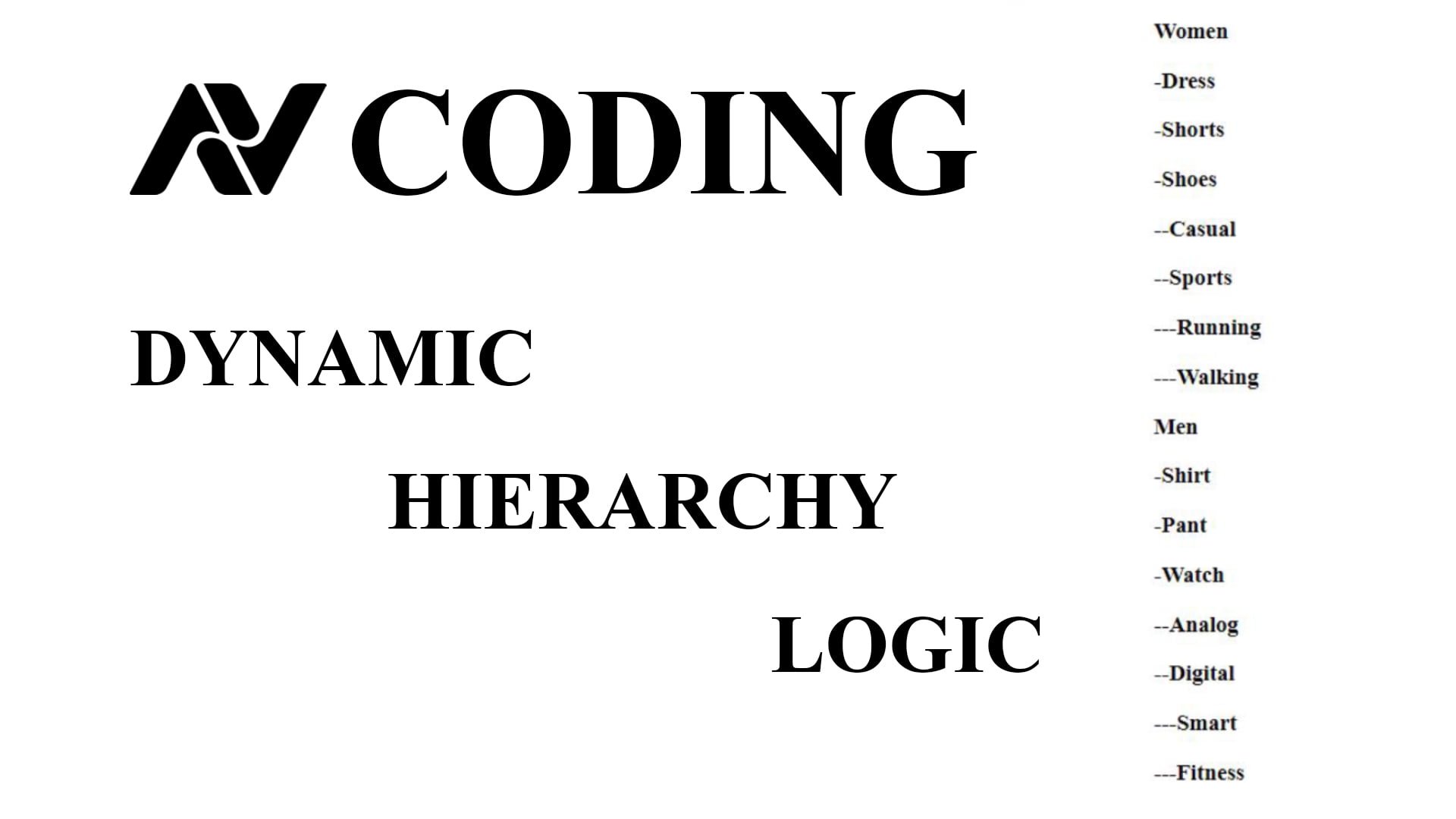
Hey guys welcome to AV Coding
Today we will learn how to handle dynamic hierarchy logic.
The complete code is explained in the video below and the source code is provided below.
<!DOCTYPE html>
<html>
<head>
<title>Dynamic Hierarchy Logic</title>
</head>
<body style = 'margin-left: 50%;'>
<h1 style = 'margin-left: -15%;'>Dynamic Hierarchy Logic</h1>
<!-- Bootstrap CDN JS Link -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script>
var data = [
{ "id": 1, "name": "Women", "hasSubCategory": true, "parentId": 0 },
{ "id": 2, "name": "Dress", "hasSubCategory": false, "parentId": 1 },
{ "id": 3, "name": "Shorts", "hasSubCategory": false, "parentId": 1 },
{ "id": 4, "name": "Shoes", "hasSubCategory": true, "parentId": 1 },
{ "id": 5, "name": "Casual", "hasSubCategory": false, "parentId": 4 },
{ "id": 6, "name": "Sports", "hasSubCategory": true, "parentId": 4 },
{ "id": 7, "name": "Running", "hasSubCategory": false, "parentId": 6 },
{ "id": 8, "name": "Walking", "hasSubCategory": false, "parentId": 6 },
{ "id": 9, "name": "Men", "hasSubCategory": true, "parentId": 0 },
{ "id": 10, "name": "Shirt", "hasSubCategory": false, "parentId": 9 },
{ "id": 11, "name": "Pant", "hasSubCategory": false, "parentId": 9 },
{ "id": 12, "name": "Watch", "hasSubCategory": true, "parentId": 9 },
{ "id": 13, "name": "Analog", "hasSubCategory": false, "parentId": 12 },
{ "id": 14, "name": "Digital", "hasSubCategory": true, "parentId": 12 },
{ "id": 15, "name": "Smart", "hasSubCategory": false, "parentId": 14 },
{ "id": 16, "name": "Fitness", "hasSubCategory": false, "parentId": 14 },
];
for(var i =0 ; i < data.length ; i++){
data[i].child = [];
for(var j = 0 ; j < data.length; j++){
if(data[i].id != data[j].id && data[i].id == data[j].parentId){
data[i].child.push(data[j]);
}
}
}
var finalData = [];
for(var i = 0; i< data.length; i++){
if(data[i].parentId == 0){
finalData.push(data[i]);
}
}
var child = [];
var spaces = '';
var br = '<br/>';
looping(finalData);
function looping(data){
for(var i = 0; i< data.length; i++){
var space = false;
if(!data[i].hasSubCategory){
if(data[i].parentId == 0){
$('body').append('<h3>'+data[i].name+'</h3>');
}else{
spacing(space, data[i]);
$('body').append('<h3>'+spaces+data[i].name+'<h3>');
spaces = spaces.slice(0,6);
}
}else{
if(data[i].parentId == 0){
child = data[i].child;
$('body').append('<h3>'+data[i].name+'<h3>');
}else{
spacing(space, data[i]);
$('body').append('<h3>'+spaces+data[i].name+'<h3>');
}
looping(data[i].child);
}
}
}
function spacing(space, data){
for(var j = 0 ; j < child.length ; j++){
if(data.id == child[j].id){
space = true;
}
}
if(!space){
spaces+= ' ';
}else{
spaces = ' ';
}
}
console.log(data);
console.log(finalData);
</script>
</body>
</html>
Hope You Liked This Blog. Share, Comment, Subscribe And Press The Bell Icon In The Bottom Right Side For More Code Feeds.
0 Comments