How To Handle Dynamic Inputs Using php & jQuery
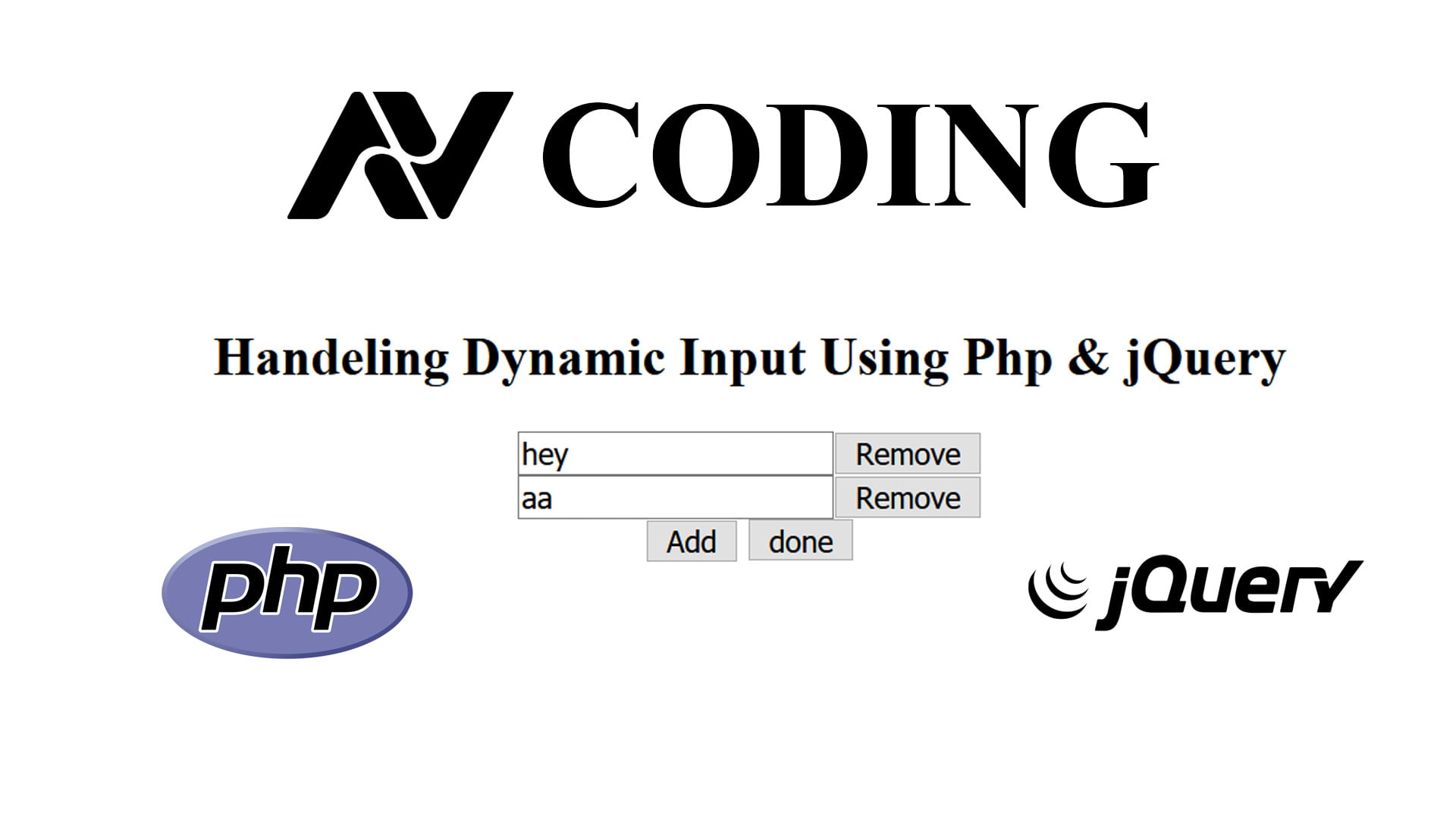
Hey guys welcome to AV Coding
Today we will learn how to handle dynamic input fields using php & jQuery.
The complete code is explained in the video below and the source code is provided below and yes don’t forget to create database and change it according to yours.
index.php
<?php
$connection = mysqli_connect('localhost', 'root', '', 'random');
if(isset($_POST['done'])){
$fields = $_GET['fields'];
$length = $fields;
$counter = 1;
if($fields >= 0){
while($length > 0){
if(isset($_POST['title'.$counter])){
$title = $_POST['title'.$counter];
if(!empty($title)){
$query = "INSERT INTO title(title)";
$query .= "VALUES('{$title}')";
$create_title = mysqli_query($connection, $query);
}
$length--;
}
$counter++;
}
$query = "SELECT * FROM title ORDER BY id ASC";
$select_title = mysqli_query($connection,$query);
while($row = mysqli_fetch_assoc($select_title)) {
$id = $row['id'];
if(isset($_POST['title'.$id])){
$title = $_POST['title'.$id];
if(empty($title)){
$query = "DELETE FROM title WHERE id = {$id} ";
$delete_title = mysqli_query($connection,$query);
}else{
$query = "UPDATE title SET ";
$query .="title = '{$title}' ";
$query .= "WHERE id = {$id} ";
$update_title = mysqli_query($connection,$query);
}
}
}
$msg = "<span class='text-success'>Diet Successfully Updated</span>";
}
if($fields < 0){
$msg = "<span class='text-danger'>Fields Error</span>";
}
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Dynamic inputs with php and jquery</title>
</head>
<body>
<h2 style="margin-top:20%;text-align:center;">Handeling Dynamic Input Using Php & jQuery</h2>
<form id='change' action="index.php?fields=0" method ='POST' style="text-align:center;">
<div id="addingdivs">
<?php
$query = "SELECT * FROM title ORDER BY id ASC";
$select_title = mysqli_query($connection,$query);
$count = 1;
while($row = mysqli_fetch_assoc($select_title)) {
$id = $row['id'];
$title = $row['title'];
?>
<input type="text" id="title<?php echo $count?>" name="title<?php echo $id?>" value="<?php echo $title;?>"><button type= "button" id="remove<?php echo $count++?>">Remove</button><br/>
<?php }?>
</div>
<button id="add" type= "button">Add</button>
<button name='done' type="submit">done</button>
</form>
<!-- jQuery CDN JS Link -->
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js" integrity="sha384-q8i/X+965DzO0rT7abK41JStQIAqVgRVzpbzo5smXKp4YfRvH+8abtTE1Pi6jizo" crossorigin="anonymous"></script>
<script>
var x = 1;
$('#add').click(function(){
$('#addingdivs').append('<input type="text" id="title'+x+'" name="title'+x+'"> <button type= "button" onclick="remove('+x+')" id="remove_temp'+x+'">Remove</button><br/>');
document.getElementById("change").action= "index.php?fields="+x;
x++;
});
function remove(id){
$("#title"+id).remove();
$("#remove_temp"+id).remove();
x--;
document.getElementById("change").action= "index.php?fields="+x;
}
<?php for($i=1 ; $i < $count; $i++){?>
$('#remove<?php echo $i;?>').click(function(){
document.getElementById("title"+$i).value = "";
});
<?php }?>
</script>
</body>
</html>
Hope You Liked This Blog. Share, Comment, Subscribe And Press The Bell Icon In The Bottom Right Side For More Code Feeds.
0 Comments